Laravel:Migration ,Models,Relations
Soit le schéma de la base de données relationelle suivante:
clients(idclient ,nom,prenom) categories(idcategorie,nom,description) produits(idproduit,#idcategorie,nom ,prix,quantiteStock,marque) vente(idvente,#idclient,#idproduit,quantite,datevente)
Créer les migrations
create_clients_table.php
php artisan make:migration create_clients_table
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
public function up()
{
Schema::create('clients', function (Blueprint $table) {
$table->id('idclient');
$table->string('nom');
$table->string('prenom');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('clients');
}
};
create_categories_table.php
php artisan make:migration create_categories_table
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
public function up()
{
Schema::create('categories', function (Blueprint $table) {
$table->id('idcategorie');
$table->string('nom');
//description text null
$table->text('description')->nullable();
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('categories');
}
};
create_produits_table.php
php artisan make:migration create_produits_table
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
public function up(): void
{
//create table produits
Schema::create('produits', function (Blueprint $table) {
//idproduit int primary key
$table->id('idproduit');
//idcategorie int
$table->bigInteger('idcategorie')->unsigned(); //idcategorie int
$table->foreign('idcategorie')->references('idcategorie')->on('categories');
//alter table add foreign key(idcategorie) references categories(idcategorie)
//nom varchar(25)
$table->string('nom');
//prix decimal(8,2)
$table->decimal('prix', 8, 2);
$table->integer('quantiteStock');
//marque varchar(25) null
$table->string('marque')->nullable();
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('produits');
}
};
create_ventes_table.php
php artisan make:migration create_ventes_table
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
public function up(): void
{
Schema::create('ventes', function (Blueprint $table) {
//idvente int primary key
$table->id('idvente');
//idclient int
$table->bigInteger("idclient")->unsigned();
//idproduit int
$table->bigInteger("idproduit")->unsigned();
//constraint foreign key(idclient) references client(idclient)
$table->foreign('idclient')->references('idclient')->on('clients'); //#idcient
//constraint foreign key(idproduit) references produit(idproduit)
$table->foreign('idproduit')->references('idproduit')->on('produits');//#idproduit
$table->integer('quantite');
$table->date('datevente');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('ventes');
}
};
Lancer la Migrations
php artisan migrate
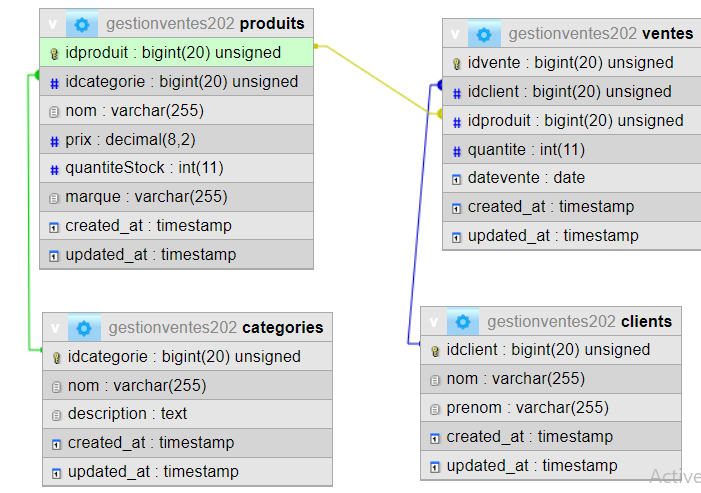
Créer les Modèles
app/Models/Client.php
php artisan make:model Client
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Client extends Model
{
// Nom de la table dans la base de données
protected $table = 'clients';
// Clé primaire de la table
protected $primaryKey = 'idclient';
// Indique si les horodatages sont utilisés (created_at et updated_at)
public $timestamps = true;
// Les attributs qui peuvent être remplis de manière massive
protected $fillable = [
'nom', 'prenom'
];
// Relation : Un client peut avoir plusieurs ventes
public function ventes()
{
return $this->hasMany('App\Models\Vente', 'idclient');
//l'attribut idclient se trouve dans le Model Vente comme un foreign key pour $primaryKey = 'idclient' dans le Model Client
}
}
app/Models/Categorie.php
php artisan make:model Categorie
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Categorie extends Model
{
protected $table = 'categories';
protected $primaryKey = 'idcategorie';
public $timestamps = true;
protected $fillable = [
'nom', 'description'
];
// Relation : Une catégorie peut avoir plusieurs produits
public function produits()
{
return $this->hasMany('App\Models\Produit', 'idcategorie');
//Model produit contient l'attribut 'idcategorie' (foreign key) qui $primaryKey = 'idcategorie' dans le Model Categorie
}
}
app/Models/Produit.php
php artisan make:model Produit
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Produit extends Model
{
protected $table = 'produits';
protected $primaryKey = 'idproduit';
public $timestamps = true;
protected $fillable = [
'idcategorie', 'nom', 'prix', 'quantiteStock', 'marque'
];
// Relation : Un produit appartient à une catégorie
public function categorie()
{
return $this->belongsTo('App\Models\Categorie', 'idcategorie');
//'attribut idcategorie dans $fillable est un foreign key du Model Categorie
}
// Relation : Un produit peut avoir plusieurs ventes
public function ventes()
{
return $this->hasMany('App\Models\Vente', 'idproduit');
// l'attribut $primaryKey = 'idproduit' se trouve comme un foreign key dans le Model Vente
}
}
app/Models/Vente.php
php artisan make:model Vente
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Vente extends Model
{
protected $table = 'ventes';
protected $primaryKey = 'idvente';
public $timestamps = true;
protected $fillable = [
'idclient', 'idproduit', 'quantite', 'datevente'
];
// Relation : Une vente appartient à un client
public function client()
{
return $this->belongsTo('App\Models\Client', 'idclient');
//l'attribut idclient dans $fillable représente un foreign key du Model Client
}
// Relation : Une vente concerne un produit
public function produit()
{
return $this->belongsTo('App\Models\Produit', 'idproduit');
//l'attribut idproduit dans $fillable représente un foreign key du Model Produit
}
}